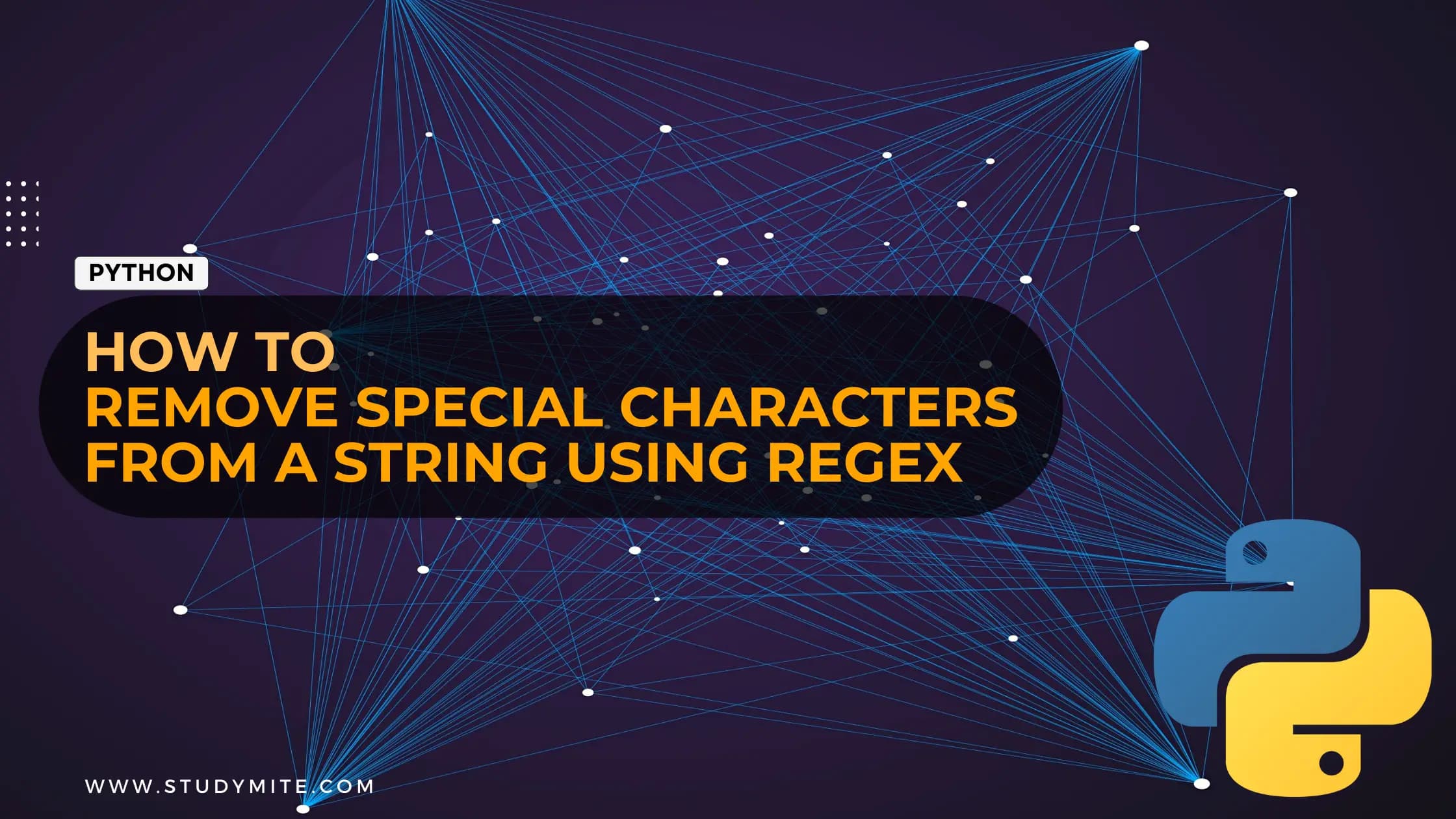
Remove Special Characters from a String in Python Using Regex
Written by
When working with strings in Python, it's not uncommon to encounter special characters such as punctuation marks, symbols, and non-printable characters. These special characters can cause issues when processing or analyzing text data and may need to be removed. One of the most efficient ways to remove special characters from a string in Python is by using regular expressions.
What is Regex?
Regular expressions, or regex for short, provide a powerful way to search and manipulate text. With regex, you can specify patterns in the text to match and then replace or remove the matched parts.
Removing special characters using Regex:
Using sub()
function:
Python's re
module provides a variety of functions that make it easy to work with regular expressions, including the sub()
function, which allows you to replace all occurrences of a pattern in a string with a replacement string.
Here's an example of how you can use the sub()
function to remove all special characters from a string:
import re
def remove_special_characters(input_string):
# Use regex to remove special characters
return re.sub('[^A-Za-z0-9]+', '', input_string)
# Test the function
original_string = 'Hello! How are you? #today'
clean_string = remove_special_characters(original_string)
print(f"Original String: {original_string}")
print(f"Clean String: {clean_string}")
It will show the following output:
Original String: Hello! How are you? #today
Clean String: HelloHowareyoutoday
In this example, the re.sub()
function replaces all occurrences of any character that is not an uppercase or lowercase letter or a digit (represented by the regular expression [^A-Za-z0-9]
) with an empty string. This means that all special characters will be removed from the input string, resulting in a clean string that contains only letters and digits. You can also use this function to remove only specific special characters by replacing [^A-Za-z0-9]
with the specific characters you want to remove.
Using translate()
method:
Another way to remove special characters from a string in Python is by using the translate()
method along with the maketrans()
method from the str
class.
Here's an example of how you can use these methods to remove specific special characters from a string:
def remove_special_characters(input_string):
translator = input_string.maketrans("", "", "!@#%^&*()_-+={}[]|\:;'<>,.?/")
return input_string.translate(translator)
# Test the function
original_string = 'Hello! How are you? #today'
clean_string = remove_special_characters(original_string)
print(f"Original String: {original_string}")
print(f"Clean String: {clean_string}")
In this example, the maketrans()
method creates a dictionary of characters which need to be replaced, the leftmost argument is the list of characters which need to be replaced, the middle argument is the list of characters with which they need to be replaced and rightmost argument is the list of characters which need to be deleted. translate()
method applies the mapping on the string, replacing special characters with nothing.
Using replace()
method:
Both sub()
and translate()
methods are very powerful in removing special characters from a string, but there are other ways to achieve this goal as well. For example, you can use the replace() method of the str class to replace specific characters with nothing, or use a list comprehension to filter out unwanted characters.
def remove_special_characters(input_string):
# use list comprehension
return "".join([c for c in input_string if c.isalnum() or c.isspace()])
This function filters out only alphanumeric and whitespace characters from the input string.
In addition, you can also use the join()
method to join a list of alphanumeric characters and whitespaces only, This method creates a new string by concatenating the elements of an iterable (such as a list or tuple) with a specified separator.
Conclusions
Ultimately, the method you choose to remove special characters from a string in Python will depend on your specific needs and the structure of your input data. Whether you use the sub() function from the re module, the translate()
method, or another approach, it's important to test your code with a variety of inputs to ensure that it's working as expected.