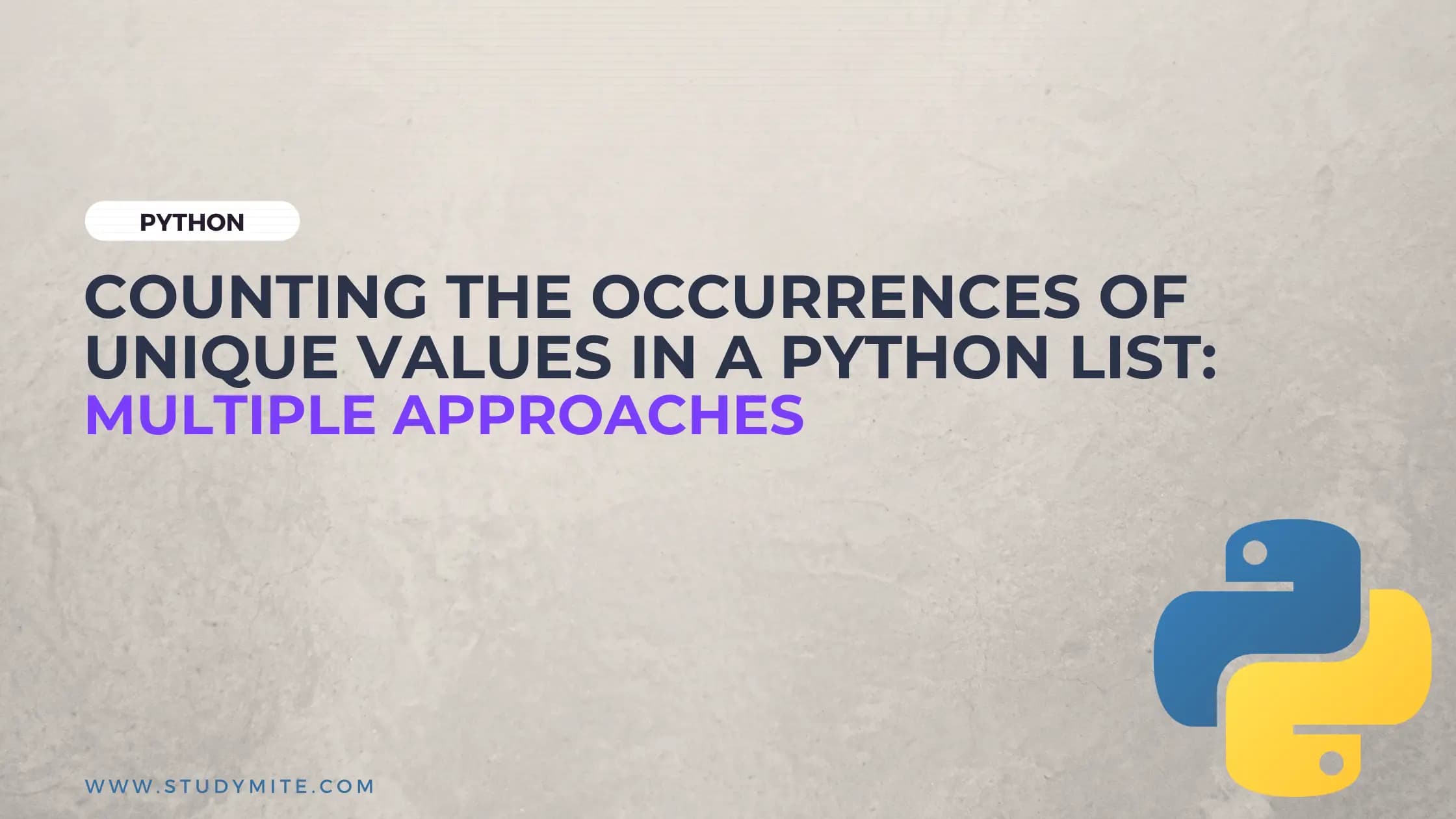
Counting the Occurrences of Unique Values in a Python List: Multiple Approaches
Written by
Counting the occurrences of unique values in a Python list is a common task that can be accomplished in multiple ways. Whether you're working with data from a survey, a log file, or a database, understanding the distribution of unique values in your data can provide valuable insights and inform your analysis.
Various ways of Counting the Occurrences of Unique Values in a Python List: Multiple Approaches
Using for
loop:
One of the simplest ways to count the occurrences of unique values in a Python list is by using a for
loop. The approach is to iterate over the list and keep a count of each unique value using a dictionary.
Here is an example:
def count_occurrences(my_list):
counts = {}
for item in my_list:
if item in counts:
counts[item] += 1
else:
counts[item] = 1
return counts
my_list = [4,3,2,1,3,1,2,3,1,4, 4,2,1, 4]
print(count_occurrences(my_list))
The output of this code will be a dictionary, whose keys are the unique values of the input list and the values are their counts, in this case:
{4: 4, 3: 3, 2: 3, 1: 4}
Using collections module:
Another way to count the occurrences of unique values in a Python list is by using the collections.Counter class from the Python standard library. The collections module provides a Counter class that takes an iterable as input and returns a dictionary with the occurrences of each unique element. Here's an example:
from collections import Counter
def count_occurrences(my_list):
return Counter(my_list)
my_list = [4,3,2,1,3,1,2,3,1,4, 4,2,1, 4]
print(count_occurrences(my_list))
The output of this code will be the same as the previous approach, but the code is more concise and recommended for large data sets.
Using count() method:
A third approach to count the occurrences of unique values in a Python list is by using a list comprehension and the count() method of the list. This approach iterates over the list to get the unique values and then uses the count() method to count their occurrences. Here's an example:
def count_occurrences(my_list):
return {x:my_list.count(x) for x in set(my_list)}
my_list = [4,3,2,1,3,1,2,3,1,4, 4,2,1, 4]
print(count_occurrences(my_list))
This approach is similar to the first one using for loop, but it is more concise and easy to read. It utilizes the set()
function which removes duplicates and then iterates over the set of unique values using a list comprehension to count the occurrences of each value in the original list using the count()
method.
Combining set and list comprehension:
Another alternative approach to count the occurrences of unique values in a Python list is using a set() and a list comprehension. Here is an example:
def count_occurrences(my_list):
return {i:my_list.count(i) for i in set(my_list)}
my_list = [4,3,2,1,3,1,2,3,1,4, 4,2,1, 4]
print(count_occurrences(my_list))
This approach uses the set()
method to remove duplicates, then iterates over the set of unique values with the list comprehension and uses the count()
method of the input list to count the occurrences.
Using numpy’s bincount()
function:
A last approach is using the numpy.bincount(x)
function from the numpy library, which counts occurrences of each unique value in the array x
.
Here is an example:
import numpy as np
def count_occurrences(my_list):
return dict(zip(np.unique(my_list),np.bincount(my_list)))
my_list = [4,3,2,1,3,1,2,3,1,4, 4,2,1, 4]
print(count_occurrences(my_list))
This approach is useful when you are already working with numpy arrays, because it's faster and more memory efficient for large datasets, but it only works for non-negative integers.
Conclusions
In conclusion, counting the occurrences of unique values in a Python list is a common task that can be accomplished in multiple ways. Whether you're working with a for loop and a dictionary, the collections.Counter class, a list comprehension and the count()
method, using a set()
and list comprehension, or the numpy.bincount(x)
function. Each approach has its own advantages and limitations, and the best method will depend on the specific needs of your program and the characteristics of your data. The for loop and dictionary approach is versatile and easy to understand for most developers, while the collections.Counter class is more concise and recommended for large data sets. The list comprehension and count()
method is easy to read and understand. Using a set()
and list comprehension is similar to the first approach but uses built-in python functions. And the numpy's bincount method is fast and memory efficient for large datasets but only works for non-negative integers.