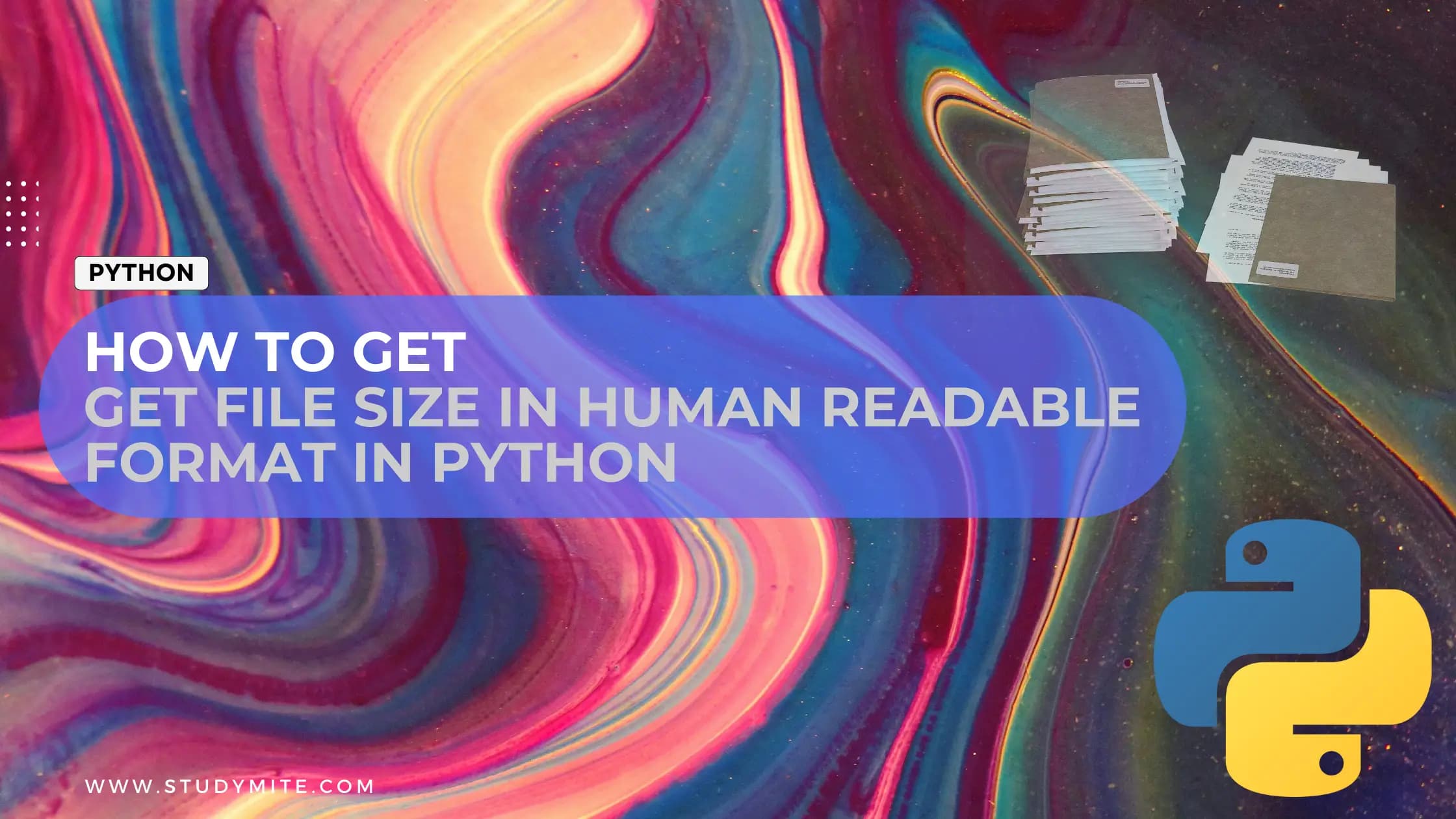
Get File Size in Human Readable Format in Python
Written by
In today's digital world, it is very common to work with files and their properties in Python, whether it is for a small project or a large application. One of the most important properties of a file is its size. Knowing the size of a file can be very useful in a number of situations, such as checking if a file meets a certain size requirement, displaying the file size to the user, or monitoring the available space on a storage device. When it comes to getting the size of a file in Python, the most common way is to retrieve it in bytes. However, it can be difficult for a user to understand the meaning of a large number of bytes. To solve this problem, the file size can be converted to a more human-readable format, such as KB, MB, GB, and TB, which is more intuitive for people to understand. In this article, we will explore various ways of getting the file size in a human-readable format using Python's built-in libraries.
Various ways of getting file size in Python
In Python, you can use the built-in libraries such as os, os.path, pathlib,shutil etc. to get the size of a file in non-human-readable format, which is typically in bytes. Here are a few examples of how you can use these libraries to get the file size in bytes:
Using the os.path
module
You can use the os.path module's getsize()
function to get the size of a file in bytes and then convert it to a human-readable format by dividing it by 1024 repeatedly and incrementing the unit index, until the file size is less than 1024 or all units have been exhausted.
For e.g.:-
import os
file_path = 'path/to/file'
file_size_bytes = os.path.getsize(file_path)
file_size_kilobytes=file_size_bytes/1000
file_size_megabytes=file_size_mega_bytes/1000
print(f"file size: {file_size_bytes} bytes")
print(f"file size: {file_size_kilobytes} kilobytes")
print(f"file size: {file_size_megabytes} megabytes")
Using os module's stat()
function:
Another way of getting the file size using the os module is using the function os.stat()
which gives the result in bytes and then convert the result to human-readable format.
For e.g.:-
import os
file_path = 'path/to/file'
file_size_bytes = os.stat(file_path).st_size
# convert the bytes to human-readable format
file_size_kilobytes=file_size_bytes/1000
file_size_megabytes=file_size_kilo_bytes/1000
print(f"file size: {file_size_bytes} bytes")
print(f"file size: {file_size_kilobytes} kilobytes")
print(f"file size: {file_size_megabytes} megabytes")
Using pathlib
library:
This is another way to get file size, you can use the method stat()
of the pathlib object on the file path and then access the st_size attribute to get the file size in bytes and then convert it to a human-readable format.
For e.g.:-
from pathlib import Path
file_path = 'path/to/file'
file_size_bytes = Path(file_path).stat().st_size
# convert the bytes to human-readable format
file_size_kilobytes=file_size_bytes/1000
file_size_megabytes=file_size_kilo_bytes/1000
print(f"file size: {file_size_bytes} bytes")
print(f"file size: {file_size_kilobytes} kilobytes")
print(f"file size: {file_size_megabytes} megabytes")
Using shutil
module:
One more way of getting the file size is by using the shutil
module's os.path.getsize()
function, it also works similar to os.path.getsize()
For e.g.:-
import shutil
file_path = 'path/to/file'
file_size_bytes = shutil.os.path.getsize(file_path)
# convert the bytes to human-readable format
file_size_kilobytes=file_size_bytes/1000
file_size_megabytes=file_size_kilo_bytes/1000
print(f"file size: {file_size_bytes} bytes")
print(f"file size: {file_size_kilobytes} kilobytes")
print(f"file size: {file_size_megabytes} megabytes")
Using subprocess
library:
Yes, you can use the subprocess
library in Python to run command-line commands like ls
or du
and extract the file size from their output. This can be useful when working with remote files and you don't have direct access to the file system, or when you need more advanced functionality that is not provided by the built-in libraries.
Here's an example of how you might use the subprocess.check_output()
function to run the ls
command and extract the file size of a remote file:
For e.g.:-
import subprocess
file_path = 'path/to/remote/file'
output = subprocess.check_output(['ls', '-l', file_path])
output_lines = output.strip().split('\n')
line = output_lines[0]
fields = line.split()
# Extract the file size from the output
file_size = fields[4]
print(f"File size of {file_path} is {file_size} bytes.")
In the above example, the subprocess.check_output()
function is used to run the ls -l
command on a remote file, which will return the file size in bytes, and the output is then parsed to extract the file size in bytes.
What is KiloByte and KibiByte:-
We often get confused with the value of KiloByte, whether it is equal to 1000 bytes or 1024 bytes. We are going to put an end to that confusion. 1 KiloByte always equals 1000 bytes.
It is 1 KibiByte which equals 1024 bytes.
Similarly, 1 MegaByte always equals 1000 KiloBytes, while 1 Mebibyte equals 1024 Kibibytes. This rule can be extended to other bigger units as well.
Conclusions
In this article, we saw various methods about how to get a file size in bytes and then convert them into human-readable format. We also got to know what KiloByte means, and what 1000 bytes or 1024 bytes is called.