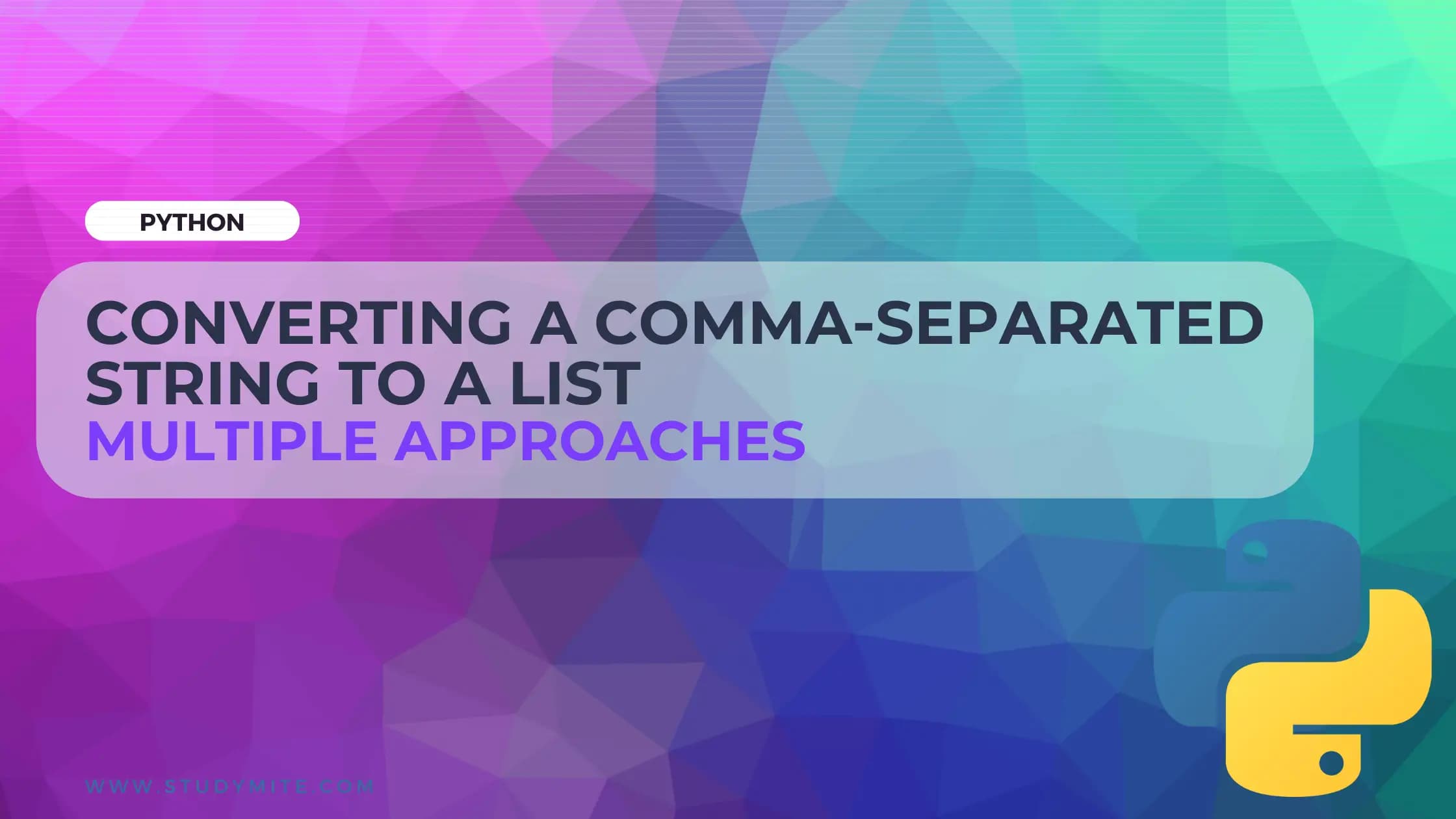
Converting a Comma-Separated String to a List in Python - Multiple Approaches
Written by
Converting a comma-separated string to a list in Python is a common task that can be accomplished in multiple ways. Whether you're working with data from a CSV file, a web form, or a database, understanding how to convert a comma-separated string to a list can provide valuable insights and inform your analysis.
Various methods of Converting a Comma-Separated String to a List in Python
Using split()
method
One of the simplest ways to convert a comma-separated string to a list in Python is by using the split() method. The split() method takes a delimiter as an argument, and returns a list of strings, with each string representing a single element from the original string, separated by the delimiter. Here is an example:
def convert_string_to_list(string):
return string.split(',')
string = "apple,banana,cherry,date"
print(convert_string_to_list(string))
The output of this code will be a list, with each element of the list being one of the items in the input string, separated by commas:
['apple', 'banana', 'cherry', 'date']
Using list
comprehension
Another way to convert a comma-separated string to a list in Python is by using a list comprehension. A list comprehension is a concise way of creating a list. This approach is useful when you are already working with string and it uses the string split()
method to make list. Here's an example:
def convert_string_to_list(string):
return [x.strip() for x in string.split(',')]
string = "apple,banana,cherry,date"
print(convert_string_to_list(string))
The output of this code will be the same as the previous approach, but it also removes leading and trailing white spaces from each element of the list, resulting in a list with clean items.
Using re
module in python
Another method to consider is using the re (regular expression) module in python. We can use the re.split()
method to split a string based on the pattern matching the delimiter.
For example, the following code uses regular expressions to split the string on the comma.
import re
def convert_string_to_list(string):
return re.split(r'\s*,\s*', string)
string = "apple, banana, cherry, date"
print(convert_string_to_list(string))
The output will be the same as previous approaches and in addition it will remove any whitespace before or after the comma, making the list elements clean.
Conclusions
In conclusion, converting a comma-separated string to a list in Python is a common task that can be accomplished in multiple ways. Some of the popular approaches include using the split()
method, using a list comprehension, and using regular expressions. Each approach has its own advantages and limitations, and the best method will depend on the specific needs of your program and the characteristics of your data. It is important to understand that some of the approaches like using list comprehension and regular expressions can give additional benefits like removing leading and trailing whitespaces. So, choosing the best approach from above depends on the input format of data which you are going to use.
Knowing how to convert a comma-separated string to a list in Python can provide valuable insights and inform your analysis.