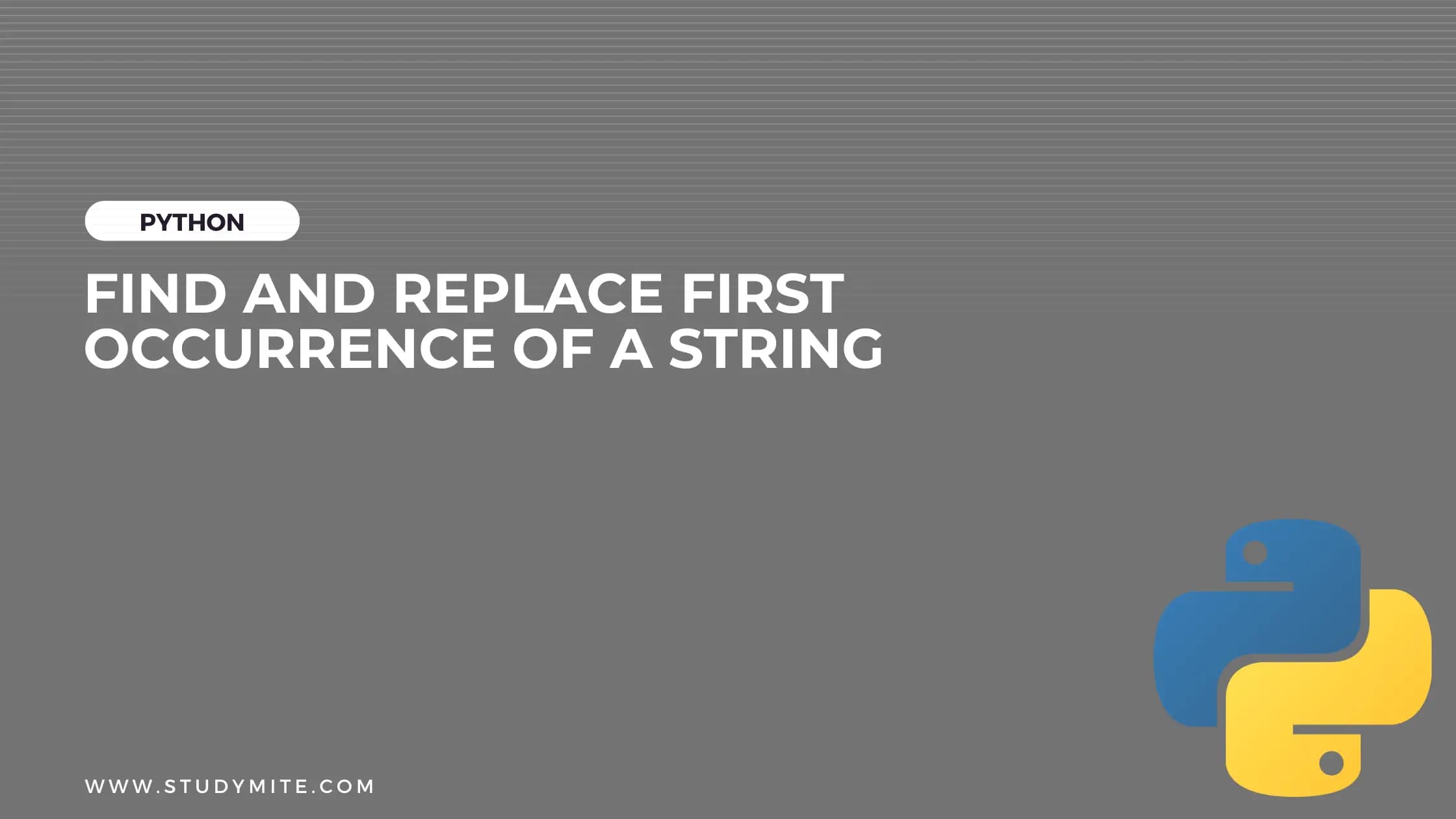
Find and Replace First Occurrence Of a String in python
Written by
In Python, there are several ways to find and replace the first occurrence of a specific word or phrase in a string.
There are various methods which one can try to achieve what is desirable.
Using replace() method:
The replace()
method, which can replace all occurrences of a specified word or phrase, or a specified number of occurrences if you pass an additional argument.
The replace()
method has the following syntax:
string.replace(old, new, count)
The old argument is the word or phrase that you want to replace. The new argument is the replacement word or phrase. The count argument is optional and specifies the number of occurrences to replace. If count is not provided, all occurrences of old will be replaced. If count is set to 1, only the first occurrence will be replaced.
Let us see an example of using replace() method to replace the first occurrence:-
string = "I have an apple and a pear."
new_string = string.replace("apple", "orange", 1)
Using find() method:
Another option is to use the find()
method to locate the position of the first occurrence of the word or phrase, and then use slicing and concatenation to create a new string with the replacement word or phrase.
The find()
method returns the index of the first occurrence of the specified word or phrase, or -1 if it is not found.
This approach is useful when you want to replace a specific word or phrase, but you don't want to use the replace()
method, which can replace all occurrences of the word or phrase by default.
Let us see an example:-
string = "I have an apple and a pear."
start = string.find("apple")
new_string = string[:start] + "orange" + string[start+5:]
Using regular expressions:
The sub()
function from the re
module can be used to find and replace the first occurrence of the word.
The sub()
function has the following syntax:
re.sub(pattern, repl, string, count=0, flags=0)
- The
pattern
argument is a regular expression that specifies the word or phrase to search for. - The
repl
argument is the replacement word or phrase. - The
string
argument is the string to search in. The ‘count’ argument is optional and specifies the number of occurrences to replace. - If
count
is not provided, all occurrences of pattern will be replaced. - If
count
is set to 1, only the first occurrence will be replaced.
This approach is useful when you want to use regular expressions to search for a specific pattern, rather than an exact word or phrase. Regular expressions allow you to search for patterns that may include wildcard characters, special characters, or specific character classes.
Let us see an example:-
import re
string = "I have an apple and a pear."
new_string = re.sub(r"apple", "orange", string, 1)
Using a for loop and slicing:
Let us begin this with an example about how this will be implemented after that we will be learning this method, by understanding the example:-
string = "I have an apple and a pear."
words = string.split()
for i, word in enumerate(words):
if word == "apple":
words[i] = "orange"
break
new_string = " ".join(words)
This code uses a for loop and the enumerate()
function to iterate over the words in the string string. The split()
method is used to split the string into a list of words, and the enumerate() function is used to keep track of the index of each word in the list.
An if statement is used to check if the current word is equal to "apple". If it is, the word is replaced with "orange" using the index, and the loop is then broken using the break statement to ensure that only the first occurrence is replaced.
Finally, the modified list of words is joined back into a single string using the join() method. The resulting string will be: "I have an orange and a pear."
This approach is useful when you want to iterate over the words in a string and perform some operation on each word. It is a more general-purpose solution that allows you to make more complex replacements based on the context or other conditions.
Conclusions:
We just saw four different ways by which we can find and replace the first occurrence of any word or phrase in a string, with each method having its own usability.