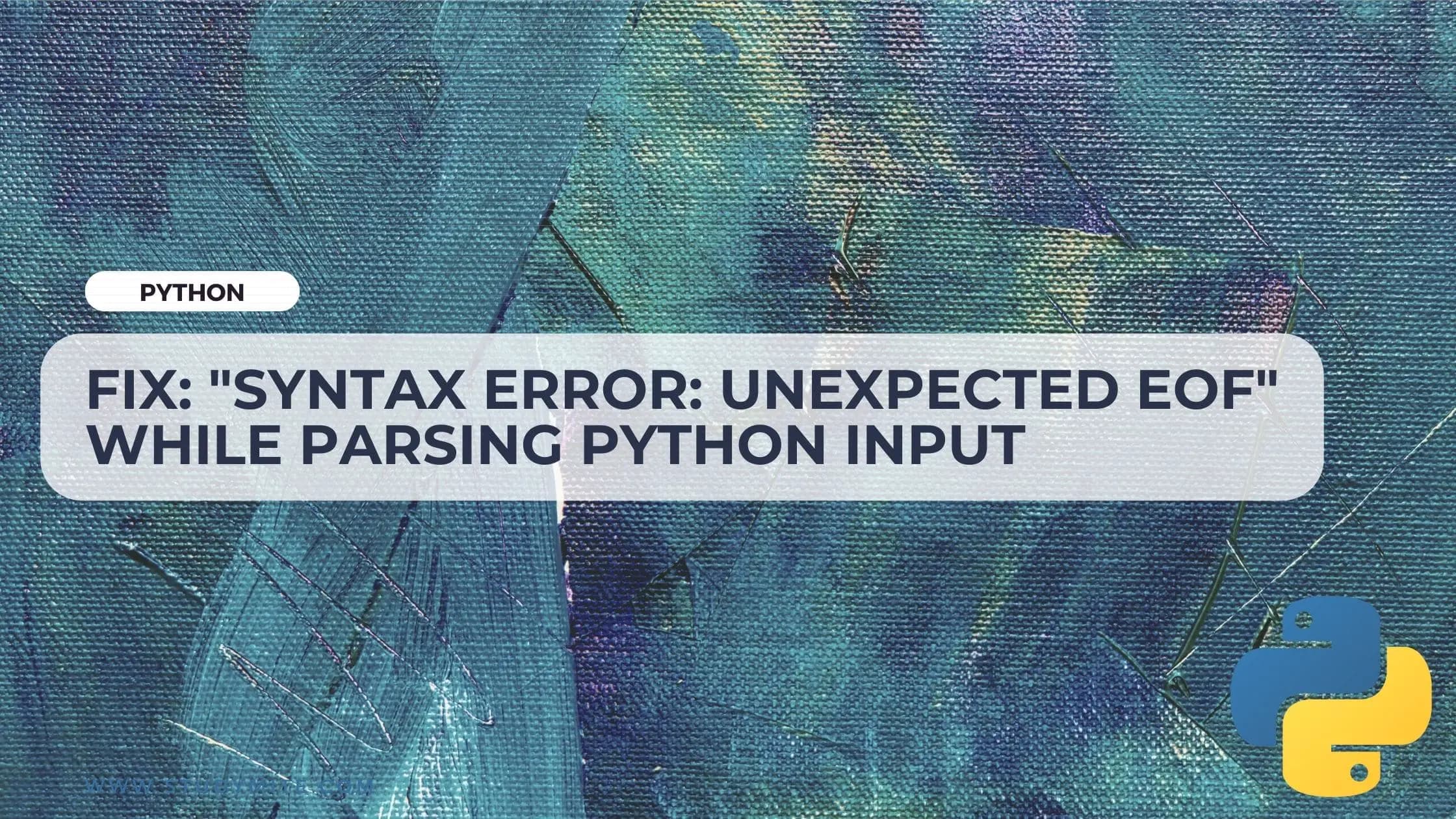
Fix: "syntax error: unexpected eof" while parsing Python input
Written by
Introduction
The "SyntaxError: unexpected EOF while parsing" error in Python occurs when the interpreter reaches the end of the input file or string and is unable to find the expected syntax. This usually means that there is a problem with the structure of your code, such as a missing parenthesis, bracket, or quotation mark.
What is Syntax Error?
In Python, a syntax error is an error that occurs when the interpreter is unable to parse a statement or expression in your code. Syntax errors are usually caused by mistakes in the structure of your code, such as incorrect indentation, missing parentheses, etc.
When a syntax error is encountered, the interpreter will raise an exception and print an error message to help you identify the problem. For example:
#Using wrong syntax for print
print “Hello World!”
It will show the below error:-
File "test.py", line 2
print "Hello World!"
SyntaxError: Missing parentheses in call to 'print'. Did you mean print("Hello World!")?
In this example, the interpreter is telling us that there is a syntax error on line 2, and it is suggesting a possible solution to the problem (adding parentheses to the print statement).
What is “syntax error: unexpected eof”?
It is a type of syntax error, when the Python interpreter is unable to find the correct syntax of the code, and thus, reached its end unexpectedly.
To fix this error, you will need to carefully review your code and look for any missing syntax elements. Here are a few common causes of this error and some suggestions for how to fix them:
- Missing closing parentheses, brackets, or curly braces: If you have opened a parentheses, bracket, or curly brace, you will need to make sure that you have a corresponding closing element. For example: ** This will cause an error because the parentheses are not closed**
def my_function(arg1, arg2
** To fix the error, make sure all parentheses, brackets, and curly braces are closed**
def my_function(arg1, arg2):
# do something
- Missing closing quotation marks: If you have opened a quotation mark, you will need to make sure that you have a corresponding closing quotation mark.
For example: This will cause an error because the string is not closed
my_string = 'hello world
To fix the error, make sure all quotation marks are closed
my_string = 'hello world'
- Mismatched quotation marks: If you have used both single and double quotation marks within the same string, you will need to make sure that the quotation marks are properly nested and match.
For example: This will cause an error because the quotation marks are mismatched
my_string = 'hello "world'
To fix the error, make sure all quotation marks are properly nested and match
my_string = "hello 'world'"
- Incomplete statements: If you have started to write a statement or expression but have not finished it, you will get an "unexpected EOF" error.
For example: This will cause an error because the assignment statement is incomplete
x =
- Unterminated multiline statements: If you begin a multiline statement like a list comprehension or a for loop) but fail to end it with a colon, you will receive a "unexpected EOF" error.
For example: This will cause an error because the list comprehension is not terminated with a colon
my_list = [x**2 for x in range(10)
- Missing closing curly braces: If you have opened a curly brace but forget to close it, you will get an "unexpected EOF" error. For example: This will cause an error because the curly braces are not closed
my_dict = {'a': 1, 'b': 2, 'c': 3,
Conclusions
To fix these types of "unexpected EOF" errors, you will need to carefully review your code and make sure that all parentheses, brackets, curly braces, quotation marks, and multiline statements are properly closed or terminated.