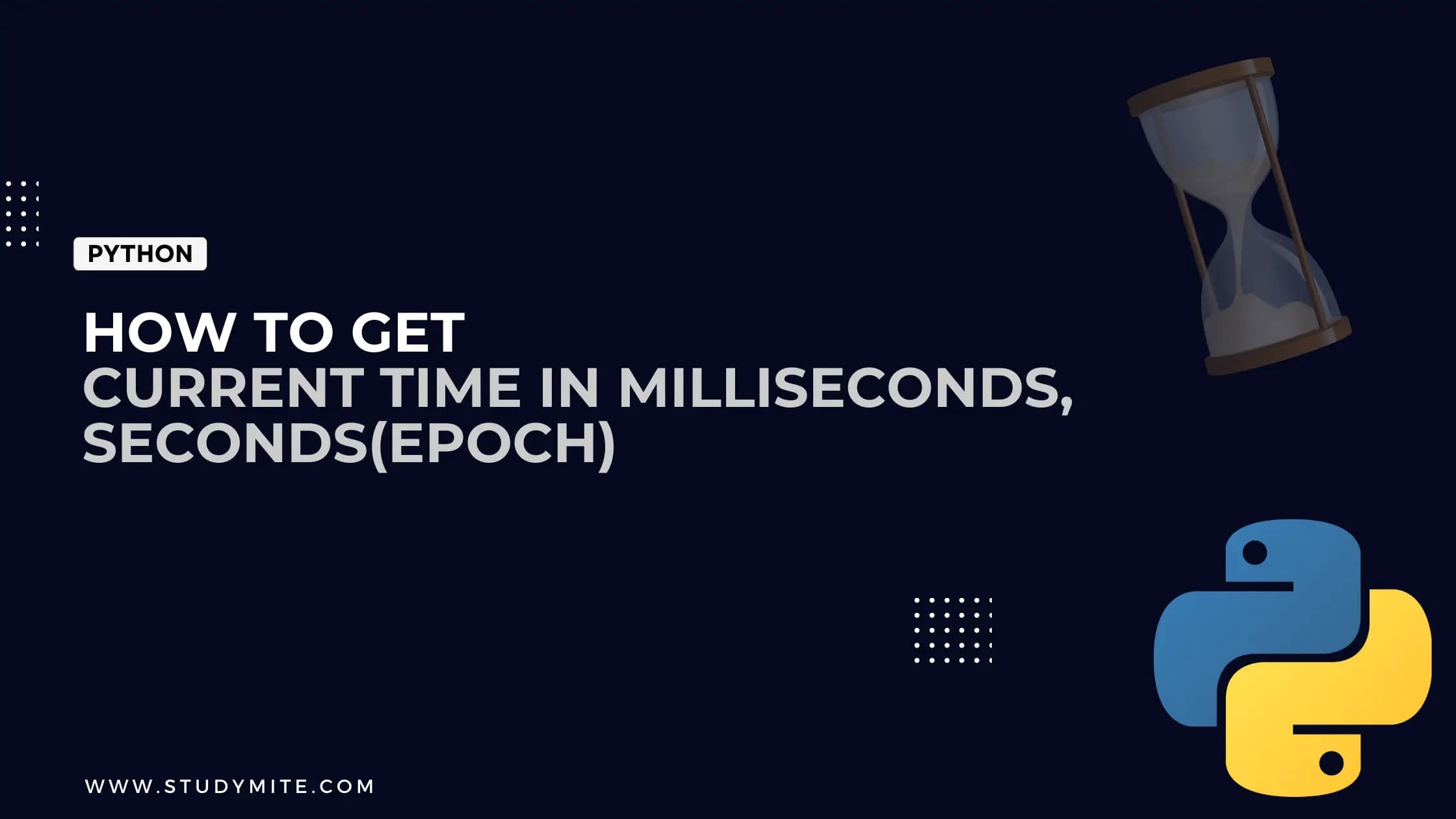
How to Get Current Time in Milliseconds, Seconds(epoch) Using Various Methods in Python
Written by
In this article, we will explore the different ways to get the current time in milliseconds and seconds (epoch) using various methods in Python.
What is an epoch?
In Python, "epoch" refers to the number of seconds that have elapsed since January 1, 1970, at 00:00:00 UTC. This date and time is known as the "epoch" or the "Unix epoch" and is used as a reference point for measuring time in many operating systems and programming languages. Python's time library gives the ability to get the time in different format than seconds, for example in seconds, milliseconds and microseconds, which can be useful in different use cases.
Various ways of calculating Current Time in Milliseconds, Seconds(epoch)
Method 1:- Using time module
import time # Get current time in seconds (epoch)
current_time_seconds = time.time()
# Get current time in milliseconds
current_time_milliseconds = int(time.time() * 1000)
The code uses the time module to get the current time in seconds (epoch) and milliseconds. The time.time()
function returns the current time in seconds since the epoch, and by multiplying the returned value by 1000, we can get the current time in milliseconds.
It's worth noting that these methods are quite simple, but it might not be the most accurate way of measuring time, especially if you need to measure time spans that are long or if you need to measure time precisely, in such cases it is recommended to use other libraries like timeit, datetime etc.
Method 2:- Using datetime module
from datetime import datetime
# Get current time in seconds (epoch)
current_time_seconds = (datetime.utcnow() - datetime(1970,1,1)).total_seconds()
# Get current time in milliseconds
current_time_milliseconds = int((datetime.utcnow() - datetime(1970,1,1)).total_seconds() * 1000)
The code uses the datetime module to get the current time in seconds (epoch) and milliseconds. This is another way to get current time in both seconds and milliseconds, the advantage of this method is that it provides the current time in UTC, which is useful in cases where time has to be represented in a universal format across different timezones.
Method 3:- Using datetime.now()
from datetime import datetime
# Get current time in seconds (epoch)
current_time_seconds = datetime.now().timestamp()
# Get current time in milliseconds
current_time_milliseconds = int(datetime.now().timestamp() * 1000)
The current time in the local timezone is obtained by calling the datetime.now()
function and its timestamp() function. This returns a float value representing the number of seconds that have elapsed since the epoch.
We can obtain current time in milliseconds by multiplying by 1000.
It gives the time in the local timezone. It's worth noting that this method does not take account of the time zone that the program is running in, so the result will be affected by the time zone difference if you are running the program in a different timezone than your system's timezone.
Method 4:- Finding current time using timeit module
import timeit
# Get current time in seconds
current_time = timeit.default_timer()
print("current time in seconds:", current_time)
In Python, the "current time" refers to the current date and time of the system that the Python script is running on. We can use the default_timer() function which provides a platform-independent way to get the current time.
Conclusions
In this article, we have discussed various ways to find the current time in seconds, milliseconds, and epoch using Python's built-in modules and functions. We have covered methods using the time module, datetime module, timeit module. By understanding the different methods available in Python, you can choose the best option for your specific use case and ensure that your code is accurate and efficient.