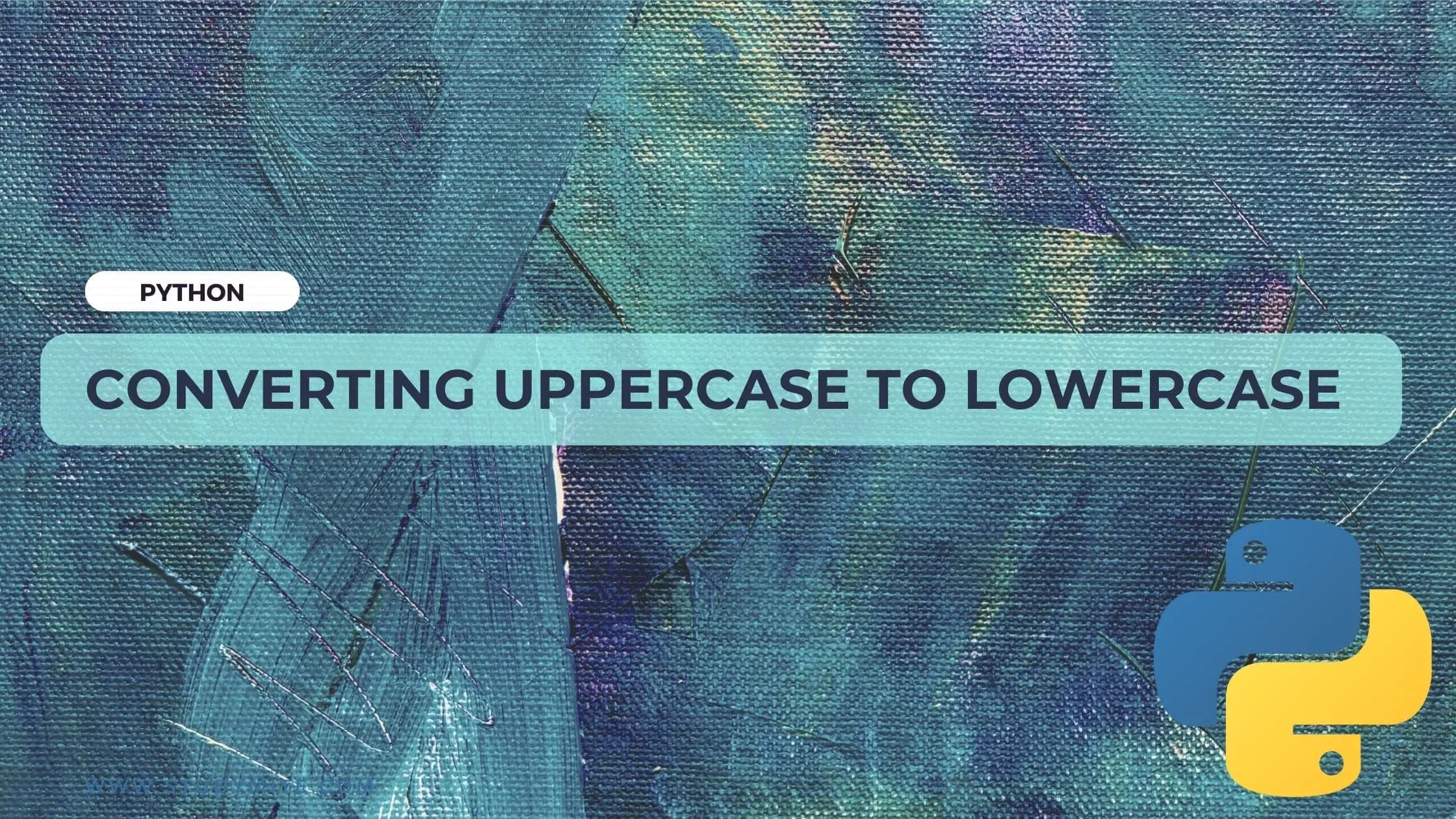
Converting Uppercase to Lowercase in Python
Written by
Converting text from uppercase to lowercase is a common task in programming, and Python provides multiple methods for achieving this without using string functions. In this article, we will explore some of the ways in which this can be done in Python.
Various methods of converting uppercase to lowercase without string functions
Using ord()
and chr()
functions
One way to convert uppercase to lowercase in Python is to use the ord()
and chr()
functions. The ord()
function returns the Unicode code point of a character, while the chr()
function returns the character that corresponds to a given Unicode code point. We can use these functions to convert uppercase characters to lowercase by adding 32 to the Unicode code point of the character. This is because uppercase characters and lowercase characters have a difference of 32 in their Unicode code points.
For e.g.:-
string = "HELLO WORLD!"
new_string = ""
for char in string:
if ord(char) in range(65, 91):
new_string += chr(ord(char) + 32)
else:
new_string += char
print(new_string)
This code snippet is using a for loop to iterate through each character in the string variable, which is initially set to "HELLO WORLD!". For each character, it checks whether its Unicode code point is within the range of 65 and 91 (inclusive) using the ord() function. This range corresponds to the uppercase letters in the ASCII character set. If the condition is true (meaning the current character is an uppercase letter), it adds 32 to the Unicode code point of the character using the ord() function, and then converts it back to a character using the chr() function. This effectively converts the uppercase character to its lowercase equivalent. The new lowercase character is then added to the new_string variable. If the condition is false (meaning the current character is not an uppercase letter), it simply adds the current character to the new_string variable without modification.
Using list comprehension and ord()
function
Another way to convert uppercase to lowercase in Python is to use list comprehension and the ord() function. This method involves creating a list of lowercase characters from an uppercase string by mapping the ord() function to each character in the string, and adding 32 to the result.
string = "HELLO WORLD!"
new_string = "".join([chr(ord(c) + 32) if 65 <= ord(c) <= 90 else c for c in string])
print(new_string)
This code snippet is using a list comprehension to convert uppercase characters to lowercase characters in the string variable, which is initially set to "HELLO WORLD!".
It uses the ord()
function to get the unicode of each character in the string and check if the unicode is between 65 and 90 (inclusive) to check if the current character is uppercase. If the current character is uppercase, it adds 32 to the unicode using the ord() function, then converts the unicode to a character using the chr() function. This effectively converts the uppercase character to its lowercase equivalent. And for the non uppercase characters it will retain the original character.
The join()
method is used to join the list of characters into a single string.
Using numpy
You can use numpy array functions to convert the text to lowercase. For e.g.:-
import numpy as np
string = "HELLO WORLD!"
new_string = np.char.lower(string)
print(new_string)
It uses the np.char.lower()
function to convert all uppercase characters in the string to lowercase. The np.char
module in numpy has several functions that operate on strings and characters. The lower()
function returns an array with all the characters of the input string converted to lowercase. Finally, the code uses the print()
function to print the new_string variable, which should contain the original string with all uppercase letters converted to lowercase. This method is good for small data and when you have large datasets that you want to perform string operations and you need performance, you can also use numpy for faster string operations,
Using pandas
You can use the str accessor on the pandas series to convert text to lowercase. For e.g.:-
import pandas as pd
string = "HELLO WORLD!"
new_string = pd.Series(string).str.lower()
print(new_string)
It first converts the string to pandas Series using pd.Series(string) , and then use .str.lower() function of the series to convert the whole series in lower case. Since a string is a sequence of characters, it's not a two dimensional data structure, so we need to convert it to a Series so that we can use the string functions. Finally, the code uses the print() function to print the new_string variable, which should contain the original string with all uppercase letters converted to lowercase.
Using regex
Python has a built-in module for working with regular expressions called re. You can use the re module to create a regular expression pattern to match uppercase letters, and then use the re.sub() function to replace them with lowercase letters. For e.g.:-
import re
string = "HELLO WORLD!"
new_string = re.sub(r'[A-Z]', lambda x: x.group(0).lower(),string)
print(new_string)
It uses the re.sub()
function to substitute all uppercase characters in the string variable with their lowercase equivalents. The first argument to the function is the regular expression pattern to match uppercase letters, r'[A-Z]', the second argument is a lambda function that uses the .group(0) method to access the matched character and convert it to lowercase by using the .lower()
method.
Finally, the code uses the print()
function to print the new_string variable, which should contain the original string with all uppercase letters converted to lowercase. This method is good for small data, but when it comes to big data, this method can be computationally expensive. Regular expressions can be relatively slow to evaluate and are not always the most efficient way to perform these types of string manipulations
Conclusions
All of the above mentioned methods are non-string methods that can be used to convert uppercase to lowercase in Python, each one is suitable for different use cases and will have different performance characteristics. It's worth noting that all the above methods can be used for small scale data, but if working with big data and performance is an issue, the string methods provided by python like lower() or casefold() will be the best choice.