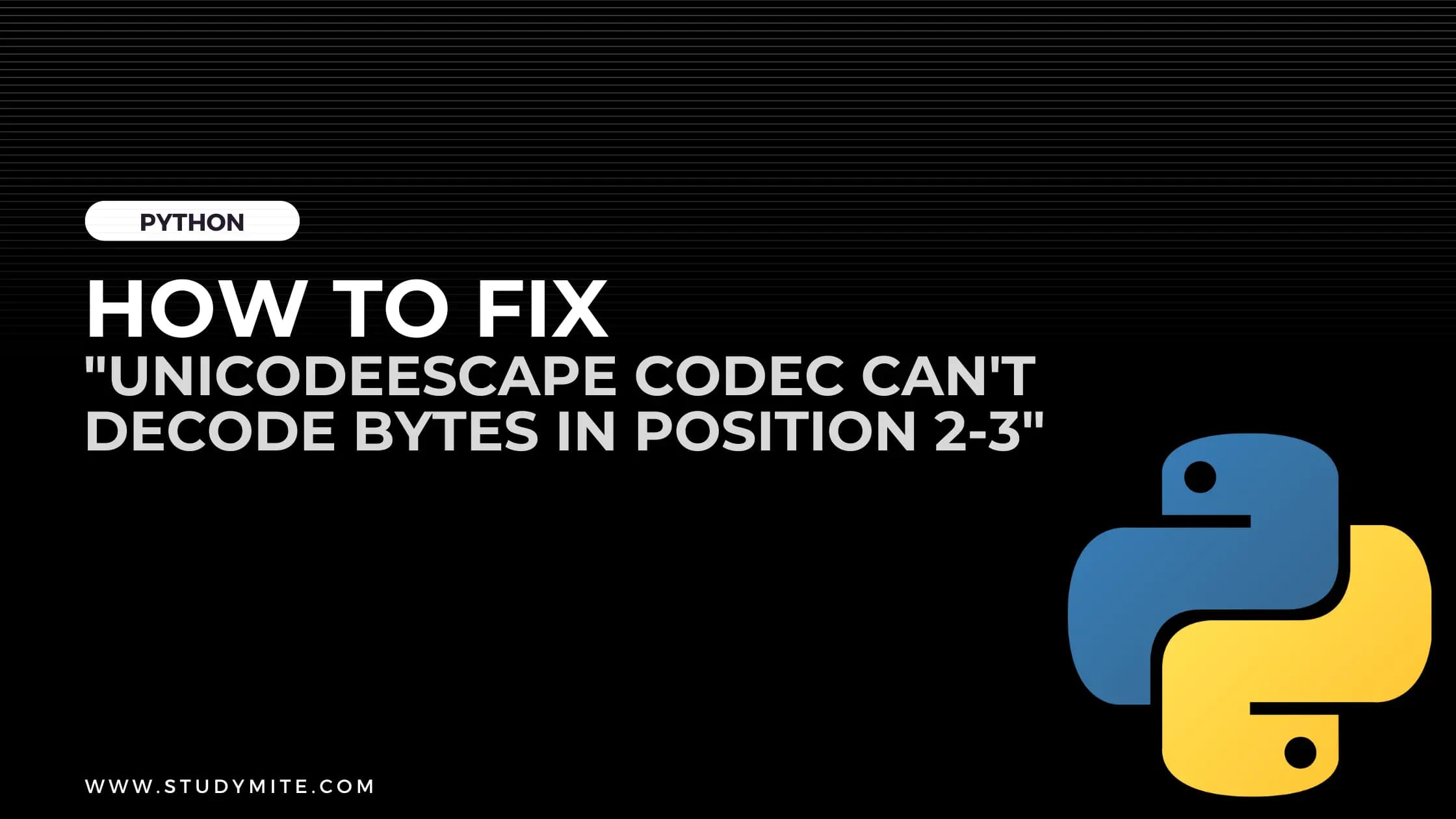
Fix: “Unicode Error: unicodeescape codec can't decode bytes in position 2-3”
Written by
Introduction
Many times we get to see "UnicodeError: UnicodeDecodeError: 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape" error in Python, and begin scratching our heads thinking about what to do next. Here, we will be seeing what this error actually means, what are its origins and how to fix it, without much effort.
What is Unicode Error in Python?
In Python, a UnicodeError is an exception that is raised when there is a problem working with Unicode strings. This can happen for a variety of reasons, such as trying to decode an invalid Unicode character or trying to encode a character that is not supported by the chosen encoding.
What is a Unicode string?
In Python, a Unicode string is a sequence of Unicode characters. Unicode is a standardized character encoding that represents most of the world's written languages. In Python, you can use Unicode strings by prefixing your string with the u character. For example:
# This is a Unicode string
my_string = u'Hello World!'
Unicode strings can contain any Unicode character, including special characters and characters from non-Latin scripts such as Chinese, Japanese, and Arabic. They are stored internally as a series of 16-bit integers, with each integer representing a Unicode code point.
Operations on Unicode String
You can use Unicode strings in Python just like you would use any other string. You can manipulate them with string methods, concatenate them with other strings, and so on. To convert a Unicode string to a regular Python string, you can use the encode method and specify an encoding such as utf-8 or latin-1. To convert a regular Python string to a Unicode string, you can use the decode method and specify an encoding. Given below is an example of encoding and decoding of a string in Python:-
# This is a Unicode string
my_string = u'Hello World!'
# Encode the string as a bytes object using the 'utf-8' encoding
my_bytes = my_string.encode('utf-8')
# Decode the bytes object back into a Unicode string using the 'utf-8' encoding
my_string = my_bytes.decode('utf-8')
Why “Unicode Error: unicodeescape codec can't decode bytes in position 2-3” error occurs?
The "UnicodeError: UnicodeDecodeError: 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape" error in Python is raised when the interpreter is trying to decode a string that contains a Unicode escape sequence, but the sequence is invalid or incomplete.
How “Unicode Error: unicodeescape codec can't decode bytes in position 2-3” error occurs and its fixes?
Invalid Unicode characters:
If you are trying to decode a string that contains an invalid Unicode character, you will get a UnicodeError. For example:
# This will cause a UnicodeError because the character '\u1234' is not a valid Unicode character
my_string = b'\x80\x81\u1234\x82'.decode('utf-8')
Unsupported encoding:
If you are trying to encode a string using an encoding that does not support certain characters, you will get a UnicodeError. For example:
# This will cause a UnicodeError because the character '\u20ac' is not supported by the 'ascii' encoding
my_string = '\u20ac'.encode('ascii')
Mismatched encoding and decoding:
If you are trying to encode a string using one encoding and then decode it using a different encoding, you may get a UnicodeError if the two encodings are incompatible. For example:
# This will cause a UnicodeError because the 'utf-8' encoding cannot decode the 'latin-1' encoding
my_string = '\u20ac'.encode('latin-1').decode('utf-8')
Invalid escape sequence:
If you have typed an invalid Unicode escape sequence, such as \U123456, the interpreter will not be able to decode it and will raise this error.
Conclusions
To fix a UnicodeError, you will need to carefully review your code and identify the cause of the error. Make sure that you are using the correct encoding and decoding functions and that your strings do not contain any invalid or unsupported characters.